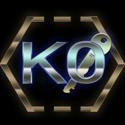
Code Injection
Code injection is a technique where you can insert code into a process and then reroute its execution to traverse through your injected custom code segment.
Working from a debugger eg. OllyDbg, we can search for “code caves”, ie. sequences of nop (or "DB 00" in Olly) instructions that are large enough to "fit" our custom code.
Alternatively we can also allocate more memory in the target process to store the code. This is what we will do in this case.
One of the best ways to inject code is via dlls, because they are meant to be loaded by multiple processes at runtime.
Compulsory ingredients:
- injector process -
DLLInjector
project - the process that will "inject" the code, - the process to inject -
ProcessToInject
project - the...