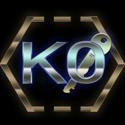
Composite Design Pattern
Join me in our quest to decipher the obscure Composite software design Pattern hieroglyphics.
Definition: Compose objects into tree structures to represent part-whole hierarchies.
The Composite pattern is a structural pattern which enables us to iterate over objects, or collection of those objects in a uniform manner, without having to typecheck or differentiate between the concrete objects and their collections. All those objects share a common interface, an abstract base class.
The whole point of the Composite pattern is that the Composite can be treated atomically as if it was a leaf object, without needing to know that there are many objects inside. Leaf objects perform a request directly, and Composite objects forward the request to their child components recursively downwards the tree...