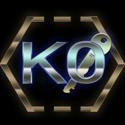
Alien Space Battle - Space Invaders lookalike Game
Want to get familiar with Python Pygame library (like I did)? Then check this "2d Space Invaders game clone" out! Pygame hides many of the down and dirty implementation details and all you're basically left to do is create the game logic. If you want to do something similar this project will surely help you.
I used Python 3.6.6, PyGame 2.0.0, Visual Studio and Windows x86_64.
File & Class Hierarchy
- images/ : directory for the texture image files
- sounds/ : directory for the sound files
- alien.py : an
Alien
class inheriting from PygameSprite
class. The constructor takes a PyGameSprite
object and sets it up. Furthermore there aredraw()
facilities (which do the rendering),update()
which updates the object's based on game logic (here it just moves it around),checkIfBottomIsReached()
tests if it has reached the bottom of the screen in which case the player loses andgameOver()
. - bullet.py : a Bullet class inheriting from Pygame Sprite class. The constructor for configuration and blitting facilities. Also
update()
moves it around. - button.py : a
Button
class. Basically just a rectangular representation of an object on the 2d ui. The constructor is used for configuration, represented with apygame.Rect
angle. Alsodraw()
andprepareMessage()
facilities. - game_functions.py : Contains all the game's functions. eg.
checkForEvents()
,startGame()
,gameOver()
,updateBackgroundImage()
,render()
everything on the screen,fireBullet()
,updateBullets()
,checkBulletAlienCollision()
etc. Everything is commented and if it's note, its code is self explanatory - this is high level Python code - come on! : ) . Adhering to a proper object oriented discipline, this should have been inside a Game class, all the functions should have been methods instead. - game_stats.py : handles saving and loading to/from the conf.ini file. The conf.ini is a .ini configuration file that persists through multiple application runs. .inis are ubiquitous in Windows programs. I hope you're aware of them, but if you're not just think of it as a sort of a save system. The .ini configuration read/store is handled by the excellent configobj module
- main.py : Starts the game up. Creates
Settings
, theShip
, the bulletsGroup()
ed, the AliensGroup()
ed, theMissile
sGroup()
ed, theStars
theScoreboard
theButton
s. Then we enter the game loop and:checkForEvents()
: Pops events frompygame.event.get()
to check screen button presses, keyboard button presses, mouse button presses, window X close etc.updateShip()
: updates the ship (ie. the player) appropriately given the user inputupdateStars()
: updates star positions, creates more of them at random positionsupdateBullets()
: updates positions for all bullets, checks collisions, removes bullets that traverse beyond screen window boundariesupdateAliens()
: updates positions of all aliens, checks if they reached the bottom of the screen, checks collisions etc.render()
: renders everything remaining on the screen
- missile.py : a
Missile
class inheriting from PygameSprite
class. The constructor for configuration and blitting facilities. Alsoupdate()
which updates its position. - scoreboard.py : a
Scoreboard
class updates the UI, scoring, highscore, the remaining ships count (ie. the lives) etc. - settings.py : a convenient
Settings
class to store our game settings. Configures PyGame initialization, lives, missiles, ships, points, stars, assets, sounds, images and more. - ship.py : a
Ship
class inheriting from PygameSprite
class. The constructor for configuration and blitting facilities. - star.py : a
Star
class inheriting from PygameSprite
class. The ctor configures its shape and appearance and adraw()
function toscreen.blit()
it to the screen
Check code comments for more information.
Horizontal / Vertical 2d Scroller game mechanics
cx_Freeze!
As an optional step, you can use cxFreeze to build an executable binary out of your Python program. To do that create a setup.py file inside the project folder AlienSpaceBattle and fill it with required options. This was mine:
#! python
#coding=utf-8
#setup.py
import os
os.environ['TCL_LIBRARY'] = "C:/Program Files/Python36/tcl/tcl8.6"
os.environ['TK_LIBRARY'] = "C:/Program Files/Python36/tcl/tk8.6"
import cx_Freeze
executables = [cx_Freeze.Executable("main.py")]
cx_Freeze.setup( name = "python_invaders",
version = "0.1",
options={"build_exe": {"packages":["pygame"],
"include_files":["images/2d-game-background-3.png","images/alien.bmp","images/alien_green.png", "images/alien_grey.png", "images/alien_nobg.tga", "images/alien_orange.png", "images/alien_purple.png", "images/destroyed_alien.png", "images/destroyed_green.png", "images/destroyed_ship.png", "images/missile.png", "images/missile_small.png", "images/raindrop.png", "images/ship.bmp", "images/ship_nobg.bmp", "images/ship_nobg.png", "images/space_background.jpg", "images/space_background.png", "images/star.png","sounds/bg_romariogrande__alien-dream.wav","sounds/fire_missile.ogg", "sounds/bg_romariogrande__alien-dream.ogg", "sounds/fire_missile.wav", "sounds/laser_bullet.wav", "sounds/laser_impact.ogg", "sounds/missile_hit.wav"]}},
description = "Space Invaders in Python. Made with PyGame by KeyC0de (Nikos Lazaridis)",
author = "KeyC0de",
executables = executables
)
Then just run it. It will create a build folder with all the assets and the executable file called main.exe
.
Controls
- P/lmb : start game
- Q : quit
- Arrow keys : move left, down, right, up
- SPACE : fire bullet
- ENTER : Fire missile
Github
Github repository link.
Acknowledgements
Sounds from Free Sounds.com. Their names indicate the author. Some don't. And I don't remember their original names as this is an old project (made circa 2016), but they were definitely on the public domain. If somebody takes issue at this I can search my download history on FreeSounds.com to find their names and list them.
Graphics mostly from me, except for the background space image which I snatched from Google public domain images. I don't remember exactly where I got it from, hence the absence of a link.