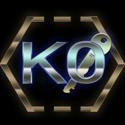
Factory Design Pattern
Definition:
Define an interface for creating an object, but let subclasses decide which class to instantiate.
Description
The factory function is used to create an object of any type of subclass of a base class.
Instead of creating objects like this:
ObjectType* o = new SubObjectType{args};
we create them like this:
ObjectType* o = newSubObjectType{args};
Where newSubObjectType
is the factory function's name. Yes, the factory is nothing more but a construction function for objects; it's typically a global/free function (not a method).
You might wonder, what is the usefulness of this design pattern?
Well, that's a legitimate question. I use the Factory pattern, when all the potential classes I'd like to create are in the same subclass hierarchy, but I don't know ahead of time (at compile time) exactly what specific class I need.
Design
- Create your class hierarchy.
- The factory function returns one of several possible classes that share a common base class.
The factory design pattern makes a, sort of, virtual constructor possible as you'll notice in the code.
Abstract Factory
The related abstract factory design pattern is again a function which is used to create an object of any type of related, or dependent subclasses. In other words it creates an object among a family of similar classes. I've never used this one but I referred to it for the sake of completion.
I used Windows and Visual Studio to build the project.
Github
Github repository link.