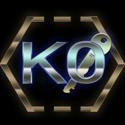
Buffer Overflow Tutorial
This is a Buffer overflow tutorial using linux Ubuntu and C programming. There is full explanation and code in Greek only at the moment in “Nikos_Lazaridis_M1485_Project#2_ΥΣ13_ΕΑΡΙΝΟ_2017.pdf”. I may be motivated to translate this in English if I see any traffic.
! During Buffer Overflow:
The ultimate goal of a Buffer overflow attack is replacing the return address of a vulnerable/unsecure function with another one of our choosing. The latter function will have "ASCII shellcode" placed into it, which will execute the attacker's desired code (WE are the attacker here); the sky's the limit with what the attacker can do (more like the imagination of the attacker). “Buffer Overflow” occurs the moment where a single byte is written in the return address of that function, in which the RIP register points to. At least that is the case in our scenario. The Instruction Pointer (IP) basically jumps to a new memory address we tell it to, from which it will resume execution.
On a higher level, there are 3 main steps in a Buffer Overflow attack (from the perspective of the attacker - us):
- create the shellcode to inject into the vulnerable function
- thoroughly examine the target program with debuggers (in Linux gdb is the standard choice and what I also used). We want to find a suitable, large enough, memory location (code cave) to place the shellcode
- also find the memory location of the vulnerable function return address through the debugger
- then infiltrate with the shellcode leading the program to transfer control to our custom injected code with arbitrary outcome
The attack has no chances of success if ASLR/DEP defense mechanisms are active, since those randomize memory segment base addresses all the time, precisely to prevent disruption from such attacks. To do this in linux Debian run the following command:
sudo echo 0 > /proc/sys/kernel/randomize_va_space
Complete memory layout of a program in Linux:
! What happens when a function in called in C?
A new stack frame has to be created. A stack frame is the memory address range between $ebp and $esp. The sequence of instructions in the stack frame correspond to the operations function.
function A:
- push function arguments
- push space for the return value
- push return address
- push fp ; (frame pointer)
- mov fp, sp ; store the stack pointer in the frame pointer
- allocate space for locals. Now the sp is decremented by one word per local variable allocated.
(jumps to the function B)
function B:
- push space for the return value
- push the address of the previous stack frame
- push values of registers that this function uses (so they can be restored later)
- push space for local variables
- perform the desired computation
- pop the registers
- pop the previous stack frame
- store the function result
- jump to the return address
(jumps back to function A)
function A:
- load the original stack pointer value which rests in the frame pointer
- pop the old frame pointer
- pop the return address
- pop the return value
Do note that:
- Branching utilizes PC-relative addressing.
- Jumps utilizes absolute addressing.
- Stack Memory "grows" towards numerically smaller memory addresses
- Stack pointer (IP) always points at the last word stored in the stack so it has a value that is always smaller than the base pointer (BP)
Stack Pointer control for inserting, removing values from a register:
addi $sp, $sp, -4 ; Subtract $sp by 4.
sw $s0, 0($sp) ; Insert value of register to stack (eg. the $s0 register)
lw $s0, ($sp) ; Remove value from the address indicated by the sp and store it in the register $s0
addi $sp, $sp, 4 ; increment $sp by 4 (to go to the next local variable's address)
Repeat these four steps for any local variables you want to extract from the stack.
ShellCode ASCII codes
|
---|
Contribute
This is distributed solely for educational purposes.
If somebody wants access to shellcodes and source zipped files send me a message and I'll send them to you. I have them zipped up with a password otherwise they will be tagged as viruses on Github and deleted (in addition to my account probably getting flagged).
Github
Github repository link.
Acknowledgements
Smashing The Stack For Fun And Profit
Phrack Magazine - Buffer Overflows