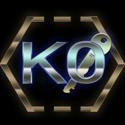
Builder Design Pattern
An introduction to the Builder software design pattern along with a fancy example, which will make you go from Zero-to-Hero in no time on becoming a master Bob the Builder!
The Builder pattern is designed to provide a flexible solution to various object creation problems in OOP. We intend to separate the construction of a complex object from its representation.
Advantages
It provides control over the individual steps in the object construction process such that we can better customize object representation. This benefit really shine when we have big complicated classes.
Disadvantages
It requires a separate Builder class for each type of such complex object and it makes dependency injection harder to work with since introducing many dependencies can get things out of hand.
Also it increases code size and almost doubles the amount of memory required since almost all variables (except possibly those with some default values and constants) have to be duplicated in the nested Builder class.
Design
- create your target big/complex class
- your target class has properties/member-variables as data. You can choose whether or not you want to provide setters/getters for them. For the sake of this example I didn't, but you totally can.
- create a nested class in it called
Builder
- The
Builder
class contains:- a default constructor - we specify its data through setter functions (more on that later)
- the state data required to build the object, which means many of the data contained in the outer/target class (if not more for data needed by other classes interacting with the target class)
- setters for each data member of the Builder class, which are called to customize the properties of our target class object. They all return a reference to
Builder
such that we can chain multiple of them nice and easy when we create the object (function chaining ftw). Alternatively you could best get rid of all setter functions in Builder & pack them all into a public data struct which you provide on create - Builder doesn't need any getters
- a
create
method returning the target Object
Usage
Create your Builder
object like so:
auto myComplexClass = ComplexClass::Builder{}
.setX(1)
.setY(2)
.setZ(3)
.setName("Key")
.setColor("Black")
.set...()
. ...
.create();
The create()
function goes last and takes no arguments.
I used Windows and Visual Studio to build the project.
Github
Github repository link.