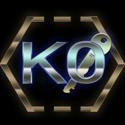
Event Queue, Message Bus and Dispatcher
NOTE: I will use the terms event and message interchangeably. In some contexts there may be a minor differentiation but for this discussion it will be ignored.
Event Queue or Message Bus
Definition:
Decouple when a message or event is sent from when it is processed. - R. Nystrom
What's the easiest way to manage the connections between event listeners and event producers? You guessed it, “Event Queues”.
When an event occurs, such as user input, or an entity sending an event to another entity, it needs to be stored somewhere such that it's not lost while in transit between the source that reported the event and when the program gets around to respond to it. That “somewhere” is a queue.
New events are added (aka enqueue
) to a queue of unprocessed events. And at a later time when it's convenient in the application we...