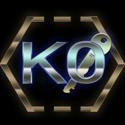
Observer Design Pattern
Observer is a behavioral software design pattern, that is being extensively utilized in almost every single self-respecting codebase as a means to efficiently communicate between objects in an event-based fashion. It wouldn't be an understatement to say it is the foundation of event based programming.
In fact, underlying the ubiquitous, in network programming, “Model View Controller” (MVC) design pattern, is the observer pattern.
Observers are used in every single game engine - they're known there mostly as “Event dispatchers”.
Alternative terminology for this pattern includes the following:
- Publish-Subscribe, Listener-Reporter.
Design
- a class, called the
Subject
, maintains a list ofObserver
s and notifies them automatically on any state changes, usually by calling one of their methods - which are typically aptly namednotify()
. - Various nosy
Observer
(concrete) classes want to know when theSubject
does something in which they are interested in and want to react to it in a potentially unique way. Those classes inherit from theIObserver
interface class. Observer
s subscribe to theSubject
class upon their construction.- In code: They are given (dependency injection) a pointer to the
Subject
, they pass it to the baseIObserver
class's constructor, and then they call theSubject
'ssubscribe
method withthis
as the argument.
- In code: They are given (dependency injection) a pointer to the
- new subscribers are added to the observer's list.
- When the subject's state, or a subset of its state that a particular observer is interested in, is changed the Subject notifies all the interested parties on this state change, by calling
notify
. - Observer objects should automatically
unsubscribe
themselves from the Subject when destroyed.
The pattern promotes loose coupling since the Subject
s don't need to know anything about the observers.
Also note that the Subject doesn't own the observers; the subject is merely referencing them. There is no need of ownership relationships in this scenario.
Homework
As a homework exercise, you can expand this project, by allowing Observers to subscribe to a particular MessageType. A MessageType is to differentiate between the actions of the subject Car. For example a Car goes forward, turns right, left, honks, crashes etc. Now one observer might not be interested in turning left or right, whereas another might only be interested in crashes (could be the insurance company : D) so it only subscribes to events of type MessageType::Crash. The misc.h
header file contains various enums/flags which can be used to improve upon. I already gave you plenty of tips to proceed on your own (and may the power protect you).
Extra Tip: for “multiple interests” the observers can pass along a bitwise OR of multiple flags to subscribe to multiple notifications at once.
I used Windows, Visual Studio and C++ 17 to build the project.
Github
Github repository link.