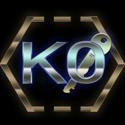
State Design Pattern
This is a demonstration of the State Design Pattern in C++ with a groovy example that will stick in your memory.
The state pattern allows an object to alter its behavior at runtime when its internal state changes. There is a base State
class and various concrete derived state classes. The state class hierarchy makes up a state machine and the desired object can be at any one single state at a point in time. State is a very similar - a structural alternative - to the Strategy pattern.
Design
- State Interface: Define an interface - abstract base class - for encapsulating the behavior associated with a particular state of the context.
- State: each concrete State - derived State class - implements the State interface's behavior. We define a fixed set of State classes that the class can be in.
- Context: maintains the data of the current state.
- the class can be in only one state at a time.
- the class starts from a default state.
- various conditions can trigger the transition from one state to another. Changing states changes the behavior of the class (at runtime).
When the object's internal state changes the object's class appears to the outside world as if it is changed and in fact it has!
Enumerations can be freely used for the selection of states. For example:
enum class State
{
STANDING,
JUMPING,
DUCKING,
DIVING,
SWIMMING
};
class Hero
{
State m_currentState;
void handleInput()
{
switch( m_currentState )
{
case State::STANDING:
// do this
break;
case State::JUMPING:
// do that
break;
// ...
default:
// do w/e (whatever)
break;
}
}
// ...
};
I used Windows and Visual Studio to build the project.
Github
Github repository link.