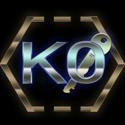
Base Friend Design Pattern
A software design pattern I've seen multiple times in codebases, that I've decided to designate (Name & Conquer). It answers the following question:
We have a certain ResourceClass
, that we wish to use its internals in various other UserClasses
. How do we achieve interaction using the base friend pattern?
It is a means to efficiently share code between different components/classes without breaking (too much) the rule of encapsulation. Classifying it as a behavioral design pattern should do nicely.
Design
We can leverage the C++ friendship rules. We're aware that C++ friends break encapsulation, "friends invade our private life". We don't like that, we hate it. However.. remember that friendship is not inherited nor bidirectional. Given this fact, we could create a base class for all our UserClasses
and that class alone can...